python数据序列集合——求交集、并集、差集
1、什么是集合
集合(set)是一个无序的不重复元素序列。
① 天生去重
② 无序
2、集合的定义
在python中,我们可以使用一对花括号{}或者set()方法来定义集合,但是如果你定义的集合是一个空集合,则只能使用set()方法。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
# 定义一个集合
s1 = {10, 20, 30, 40, 50}
print(s1)
print(type(s1))
# 定义一个集合:集合中存在相同的数据
s2 = {'刘备', '曹操', '孙权', '曹操'}
print(s2)
print(type(s1))
# 定义空集合
s3 = {}
s4 = set()
print(type(s3)) # <class 'dict'>
print(type(s4)) # <class 'set'>
|
3、集合操作的相关方法(增删查)
☆ 集合的增操作
① add()方法:向集合中增加一个元素(单一)
1
2
3
4
|
students = set()
students.add('李哲')
students.add('刘毅')
print(students)
|
② update()方法:向集合中增加序列类型的数据(字符串、列表、元组、字典)
1
2
3
4
|
students = set()
list1 = ['刘备', '关羽', '赵云']
students.update(list1)
print(students)
|
1
2
3
4
5
|
students = set()
students.add('刘德华')
students.add('黎明')
# 使用update新增元素
students.update('蔡徐坤')
|
☆ 集合的删操作
① remove()方法:删除集合中的指定数据,如果数据不存在则报错。
② discard()方法:删除集合中的指定数据,如果数据不存在也不会报错。
③ pop()方法:随机删除集合中的某个数据,并返回这个数据。
1
2
3
4
5
6
7
8
9
10
11
|
# 1、定义一个集合
products = {'萝卜', '白菜', '水蜜桃', '奥利奥', '西红柿', '凤梨'}
# 2、使用remove方法删除白菜这个元素
products.remove('白菜')
print(products)
# 3、使用discard方法删除未知元素
products.discard('玉米')
print(products)
# 4、使用pop方法随机删除某个元素
del_product = products.pop()
print(del_product)
|
☆ 集合中的查操作
① in :判断某个元素是否在集合中,如果在,则返回True,否则返回False
② not in :判断某个元素不在集合中,如果不在,则返回True,否则返回False
1
2
3
4
5
6
7
|
# 定义一个set集合
s1 = {'刘帅', '英标', '高源'}
# 判断刘帅是否在s1集合中
if '刘帅' in s1:
print('刘帅在s1集合中')
else:
print('刘帅没有出现在s1集合中')
|
③ 集合的遍历操作
1
2
|
for i in 集合:
print(i)
|
4、集合中的交集、并集与差集特性
在Python中,我们可以使用&
来求两个集合的交集:
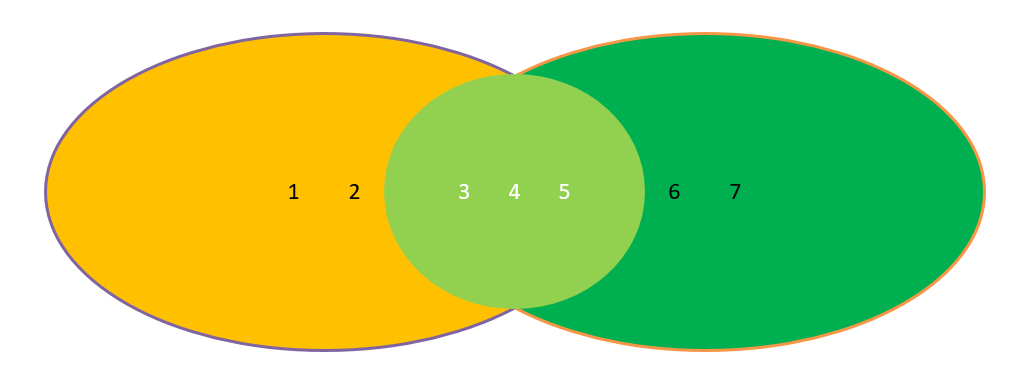
在Python中,我们可以使用|
来求两个集合的并集:
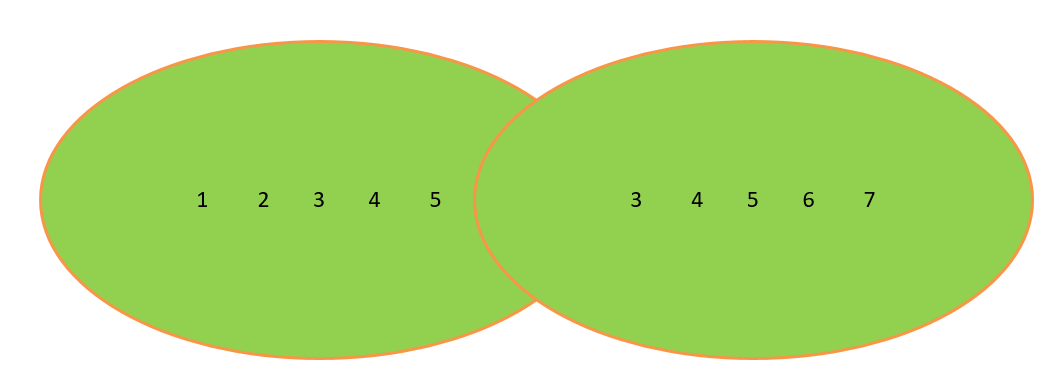
在Python中,我们可以使用-
来求两个集合的差集:
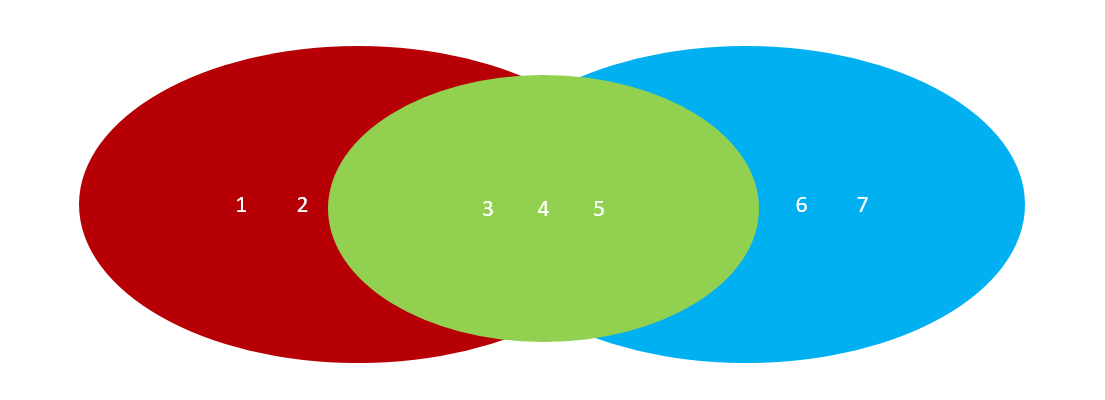
1
2
3
4
5
6
7
8
9
10
11
12
13
|
# 求集合中的交集、并集、差集
s1 = {'刘备', '关羽', '张飞', '貂蝉'}
s2 = {'袁绍', '吕布', '曹操', '貂蝉'}
# 求两个集合中的交集
print(s1 & s2)
# 求两个集合中的并集
print(s1 | s2)
# 求连个集合中的差集
print(s1 - s2)
print(s2 - s1)
|